Polars: Empowering Large-Scale Data Analysis in Python
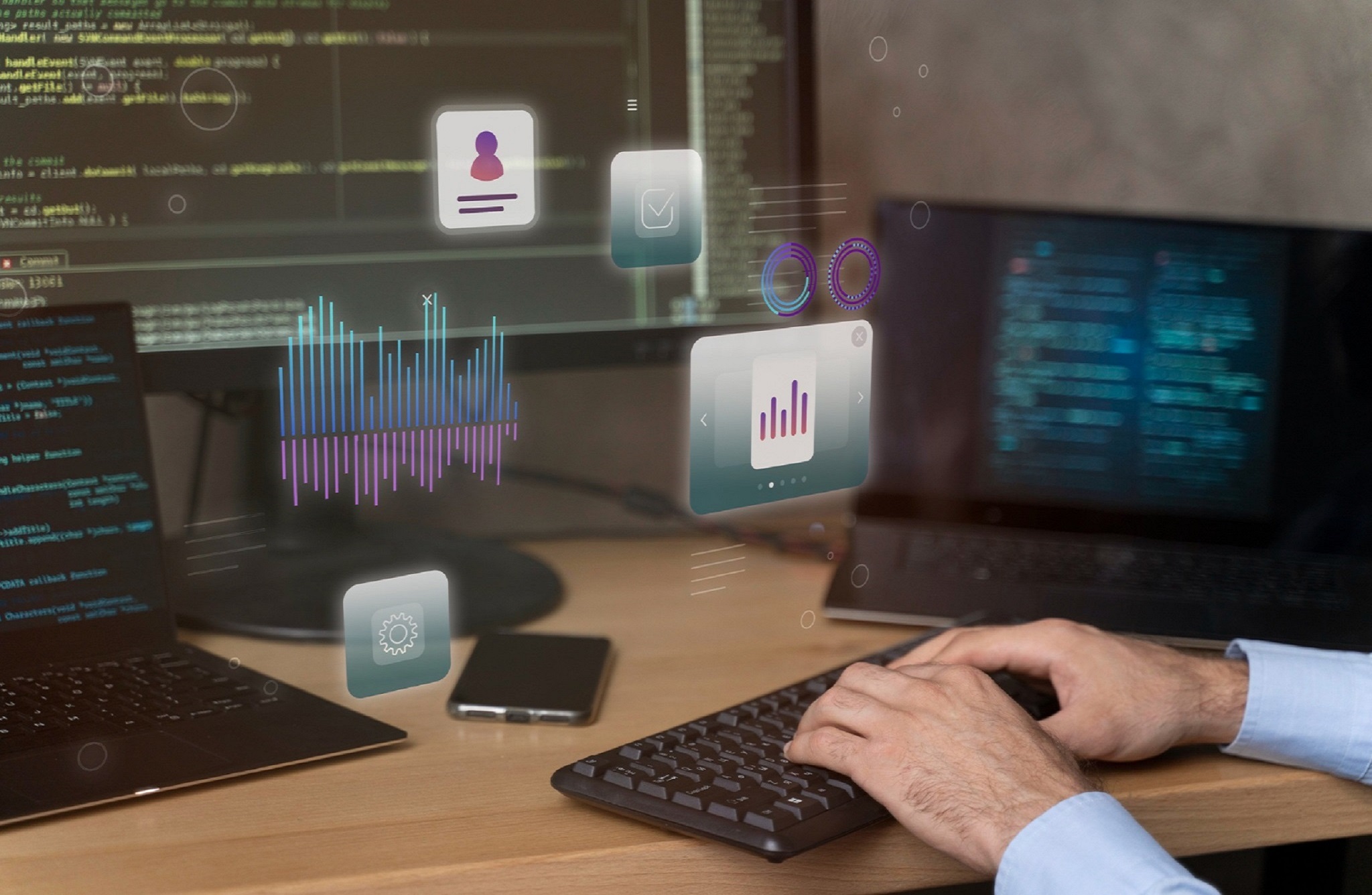
In today’s data-driven world, analyzing vast datasets efficiently is a crucial aspect of decision-making and problem-solving. Python, a versatile programming language, offers various libraries and tools for data manipulation and analysis. One such powerful tool is Polars. Efficiently managing extensive datasets demands tools capable of fast computations and streamlined operations. This is precisely where Polars excels. Polars stands as a potent open-source library tailored to excel in high-performance data manipulation and analysis within the Python ecosystem.
In this article, we will delve into Polars, exploring its features, advantages, and how it can help you handle extensive datasets effortlessly.
Table Of Contents
1. What are polars ?
Polars is an open-source data manipulation and analysis library for Python. It is designed to handle large-scale data with ease, making it an excellent choice for data engineers, data scientists, and analysts dealing with extensive datasets. Polars provides a high-level API that simplifies data operations, making it accessible to both beginners and experienced professionals.
2. Comparing Polars with Pandas
Lazy Evaluation vs. In-Memory Processing:
- Polars: Employs a lazy evaluation strategy, processing data step by step, which allows it to handle datasets larger than the available memory.
- Pandas: Loads entire datasets into memory, making it less suitable for large datasets that may exceed available RAM.
Parallel Execution:
- Polars: Leverages parallel execution to process data efficiently by distributing computations across multiple CPU cores.
- Pandas: Primarily relies on single-threaded execution, which can lead to performance bottlenecks with large datasets.
Performance with Large Datasets:
- Polars: Excels at handling large datasets efficiently and delivers impressive performance even as dataset sizes grow.
- Pandas: May suffer from extended processing times as dataset sizes increase, potentially limiting productivity.
Ease of Learning:
- Polars: Offers a user-friendly API that is easy to learn, making it accessible to both beginners and experienced data professionals.
- Pandas: are known for their flexibility but may have a steeper learning curve, especially for newcomers to data analysis.
Integration with Other Libraries:
- Polars: Seamlessly integrates with various Python libraries, including Matplotlib, Seaborn, and Plotly, for advanced visualization and analysis.
- Pandas: Also supports integration with external libraries but may require more effort for seamless collaboration.
Advanced Visualization:
- Polars: Provides basic data visualization capabilities but is primarily focused on data manipulation and analysis.
- Pandas: Offers more extensive visualization options, making it suitable for creating a wide range of plots and charts.
Memory Efficiency:
- Polars: Prioritizes memory efficiency by avoiding unnecessary data loading, which can be advantageous for systems with limited RAM.
- Pandas: Loads entire datasets into memory, which can be resource-intensive.
3. Polars Installation
Installing Polars is a straightforward process. You can follow these steps to install Polars in your Python environment:
Using pip:
Open your terminal or command prompt and run the following command:
pip install polars
This command will download and install the Polars library and its dependencies. Make sure your Python environment is properly set up before running this command.
Using Conda (if you prefer Conda for package management):
You can also install Polars using Conda by running the following command:
conda install -c conda-forge polars
import polars as pl
Now you’re ready to explore the powerful features of Polars for working with data in Python.
4. Features of Polars
Data Loading and Storage
CSV (Comma-Separated Values): CSV files are one of the most common ways to store structured data. Polars allows you to read and write data from and to CSV files effortlessly, making it easy to work with tabular data.
Parquet: Parquet is a columnar storage file format known for its efficiency and compatibility with big data processing frameworks like Apache Spark. Polars provides robust support for Parquet files, enabling efficient data access and manipulation.
Arrow: Apache Arrow is an open-source, in-memory data format that promotes interoperability between different data processing tools. Polars can seamlessly interface with Arrow data structures, facilitating data interchange and compatibility with Arrow-enabled ecosystems.
JSON (JavaScript Object Notation): JSON is a widely used format for semi-structured and nested data. Polars can read and process JSON files, making it suitable for working with data in this format.
SQL Databases: Polars offers the capability to connect to SQL databases directly. This feature simplifies data retrieval and analysis from relational databases like SQLite, PostgreSQL, and MySQL, allowing you to run SQL queries on your data.
DataFrames: Polars is designed to work efficiently with its native data structures, known as DataFrames. This means you can easily create DataFrames within your Python environment and leverage Polars’ features for data manipulation and analysis.
URLs and HTTP Endpoints: Polars enables the retrieval of data from remote sources, such as web URLs and HTTP endpoints. This capability is valuable for real-time data analysis and integration with web-based data streams.
Custom Data Sources: For specialized use cases, Polars provides the flexibility to define custom data sources and connectors. This feature allows you to work with data in unique and proprietary formats, making Polars adaptable to various data scenarios.
Data Loading and Storage
CSV (Comma-Separated Values): CSV files are one of the most common ways to store structured data. Polars allows you to read and write data from and to CSV files effortlessly, making it easy to work with tabular data.
Parquet: Parquet is a columnar storage file format known for its efficiency and compatibility with big data processing frameworks like Apache Spark. Polars provides robust support for Parquet files, enabling efficient data access and manipulation.
Arrow: Apache Arrow is an open-source, in-memory data format that promotes interoperability between different data processing tools. Polars can seamlessly interface with Arrow data structures, facilitating data interchange and compatibility with Arrow-enabled ecosystems.
JSON (JavaScript Object Notation): JSON is a widely used format for semi-structured and nested data. Polars can read and process JSON files, making it suitable for working with data in this format.
SQL Databases: Polars offers the capability to connect to SQL databases directly. This feature simplifies data retrieval and analysis from relational databases like SQLite, PostgreSQL, and MySQL, allowing you to run SQL queries on your data.
DataFrames: Polars is designed to work efficiently with its native data structures, known as DataFrames. This means you can easily create DataFrames within your Python environment and leverage Polars’ features for data manipulation and analysis.
URLs and HTTP Endpoints: Polars enables the retrieval of data from remote sources, such as web URLs and HTTP endpoints. This capability is valuable for real-time data analysis and integration with web-based data streams.
Custom Data Sources: For specialized use cases, Polars provides the flexibility to define custom data sources and connectors. This feature allows you to work with data in unique and proprietary formats, making Polars adaptable to various data scenarios.
Loading Data from a CSV File:
import polars as pl
# Define the file path to your CSV data
csv_file_path = “your_data.csv”
# Load the CSV data into a Polars DataFrame
df = pl.read_csv(csv_file_path)
# Display the first few rows of the data frame.
print(df.head())
In the code above:
- We import the Polars library as pl.
- Specify the path to your CSV file in the csv_file_path variable.
- Use pl.read_csv() to load the data from the CSV file into a Polars DataFrame named df.
- Finally, we display the first few rows of the DataFrame using df.head().
Saving Data to a CSV File:
# Define the file path for saving the DataFrame as a CSV file
output_csv_file = “output_data.csv”
# Save the DataFrame to a CSV file
df.write_csv(output_csv_file)
# Optionally, you can specify options like delimiter and header
# df.write_csv(output_csv_file, delimiter=’,’, with_headers=True)
In this part of the code:
- We specify the file path where we want to save the DataFrame as a CSV file using the output_csv_file variable.
- Use df.write_csv(output_csv_file) to save the DataFrame as a CSV file. You can also provide additional options like specifying the delimiter and including headers if needed, as shown in the commented line.
Data Transformation and Manipulation
1. Data Filtering:
Data filtering allows you to extract specific rows from a DataFrame based on conditions. Here’s how you can do it with Polars:
import polars as pl
# Create a sample DataFrame
data = {‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’, ‘David’],
‘Age’: [25, 30, 22, 35]}
df = pl.DataFrame(data)
# Filter rows where Age is greater than 25
filtered_df = df.filter(df[‘Age’] > 25)
print(filtered_df)
In this example, filtered_df will contain only the rows where the ‘Age’ column is greater than 25.
2.Data Aggregation:
Data aggregation allows you to compute summary statistics or perform operations on groups of data. Here’s how to aggregate data with Polars:
# Create a sample DataFrame
data = {‘Category’: [‘A’, ‘B’, ‘A’, ‘B’, ‘A’],
‘Value’: [10, 15, 20, 25, 30]}
df = pl.DataFrame(data)
# Group by ‘Category’ and calculate the sum of ‘Value’ for each group
agg_df = df.groupby(‘Category’).agg(pl.sum(‘Value’).alias(‘Total_Value’))
print(agg_df)
In this example, agg_df will show the total sum of ‘Value’ for each unique ‘Category’.
3.Data Joining:
Data joining allows you to combine information from multiple DataFrames based on common columns. Here’s how you can join DataFrames with Polars:
# Create two sample DataFrames
data1 = {‘ID’: [1, 2, 3],
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’]}
data2 = {‘ID’: [2, 3, 4],
‘Salary’: [50000, 60000, 70000]}
df1 = pl.DataFrame(data1)
df2 = pl.DataFrame(data2)
# Perform an inner join based on the ‘ID’ column
joined_df = df1.join(df2, on=’ID’, how=’inner’)
print(joined_df)
In this example, joined_df will contain only the rows where the ‘ID’ column matches in both DataFrames, combining information from both tables.
Polars provides a concise and expressive API for these and many other data transformation tasks, making it a powerful tool for data manipulation and analysis in Python.
Integration with Other Libraries
Polars seamlessly integrates with various Python libraries, enhancing its capabilities for data analysis and visualization. Here’s how you can integrate Polars with popular libraries like Matplotlib, Seaborn, and Jupyter notebooks:
- Integration with Matplotlib:
Matplotlib is a widely-used library for creating static, animated, and interactive visualizations in Python. You can use Polars in conjunction with Matplotlib to create insightful plots and charts based on your data.
import polars as pl
import matplotlib.pyplot as plt
# Create a sample Polars DataFrame
data = {‘Month’: [‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’],
‘Sales’: [1000, 1200, 800, 1500]}
df = pl.DataFrame(data)
# Convert the Polars DataFrame to a Pandas DataFrame
df_pandas = df.to_pandas()
# Create a bar plot using Matplotlib
plt.bar(df_pandas[‘Month’], df_pandas[‘Sales’])
plt.xlabel(‘Month’)
plt.ylabel(‘Sales’)
plt.title(‘Monthly Sales’)
# Display the plot
plt.show()
In this example, we convert a Polars DataFrame to a Pandas DataFrame (df_pandas) and then use Matplotlib to create a bar plot. This seamless integration allows you to leverage the data manipulation capabilities of Polars along with the visualization power of Matplotlib.
2. Integration with Seaborn:
Seaborn is a statistical data visualization library that works well with Pandas DataFrames. You can combine Polars and Seaborn to create aesthetically pleasing and informative visualizations.
import polars as pl
import seaborn as sns
import matplotlib.pyplot as plt
# Create a sample Polars DataFrame
data = {‘Category’: [‘A’, ‘B’, ‘A’, ‘B’, ‘A’],
‘Value’: [10, 15, 20, 25, 30]}
df = pl.DataFrame(data)
# Convert the Polars DataFrame to a Pandas DataFrame
df_pandas = df.to_pandas()
# Create a Seaborn boxplot
sns.boxplot(x=’Category’, y=’Value’, data=df_pandas)
# Display the plot
plt.show()
In this example, we convert the Polars DataFrame to a Pandas DataFrame and then use Seaborn to create a boxplot. The seamless interaction between Polars and Seaborn allows you to leverage Polars for data preprocessing and Seaborn for advanced visualization.
3. Integration with Jupyter Notebooks:
Polars can be seamlessly integrated with Jupyter notebooks, providing an interactive environment for data analysis. You can install Polars in your Jupyter environment and use it alongside other Jupyter-friendly libraries.
# Install Polars in Jupyter Notebook
!pip install polars
import polars as pl
import matplotlib.pyplot as plt
# Create and manipulate Polars DataFrames as needed
data = {‘Month’: [‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’],
‘Sales’: [1000, 1200, 800, 1500]}
df = pl.DataFrame(data)
# Visualize data using Matplotlib within the Jupyter notebook
plt.plot(df[‘Month’], df[‘Sales’])
plt.xlabel(‘Month’)
plt.ylabel(‘Sales’)
plt.title(‘Monthly Sales’)
# Display the plot within the notebook
plt.show()
In a Jupyter notebook, you can directly install Polars, create and manipulate Polars DataFrames, and visualize data using libraries like Matplotlib or Seaborn. The interactivity of Jupyter notebooks complements Polars’ data analysis capabilities.
Visualizations with Polars
Polars, primarily designed for data manipulation and analysis, also offers basic data visualization capabilities. While it may not provide as extensive visualization features as dedicated libraries like Matplotlib or Seaborn, you can still create simple visualizations directly within Polars. Let’s explore some of the basic visualization options available in Polars:
1. Line Plot:
You can create a line plot to visualize the trends or changes in data over time or across categories.
import polars as pl
import matplotlib.pyplot as plt
# Create a sample Polars DataFrame
data = {‘Month’: [‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’],
‘Sales’: [1000, 1200, 800, 1500]}
df = pl.DataFrame(data)
# Create a line plot using Polars
df.plot(x=’Month’, y=’Sales’, kind=’line’)
# Display the plot
plt.show()
2. Bar Plot:
Bar plots are useful for comparing data across categories.
# Create a bar plot using Polars
df.plot(x=’Month’, y=’Sales’, kind=’bar’)
# Display the plot
plt.show()
3. Histogram:
Histograms help you visualize the distribution of data.
# Create a histogram using Polars
df.plot(x=’Sales’, kind=’hist’)
# Display the plot
plt.show()
4. Scatter Plot:
Scatter plots are effective for visualizing relationships between two variables.
# Create a scatter plot using Polars
data = {‘Height’: [160, 165, 170, 175, 180],
‘Weight’: [55, 60, 65, 70, 75]}
df = pl.DataFrame(data)
df.plot(x=’Height’, y=’Weight’, kind=’scatter’)
# Display the plot
plt.show()
5. Box Plot:
Box plots help in understanding the distribution and spread of data.
# Create a box plot using Polars
data = {‘Category’: [‘A’, ‘B’, ‘A’, ‘B’, ‘A’],
‘Value’: [10, 15, 20, 25, 30]}
df = pl.DataFrame(data)
df.plot(x=’Category’, y=’Value’, kind=’box’)
# Display the plot
plt.show()
5. Conclusion For Polars
In conclusion, Polars is a powerful and versatile library for data manipulation and analysis in Python. It offers several notable advantages, including:
- Efficient handling of large datasets
- Lazy evaluation for memory optimization
- Seamless integration with other Python libraries
- An easy-to-learn API
Polars empowers data professionals, data scientists, and analysts to streamline their data analysis workflows, uncover valuable insights, and make data-driven decisions effectively. Its ability to work with various data sources, support for data transformation operations, and basic visualization capabilities make it a valuable tool in the data analysis toolkit.
While Polars may not replace dedicated visualization libraries, its ability to work in tandem with them allows users to combine the strengths of both data manipulation and visualization to create comprehensive data analysis solutions.
As the data landscape continues to evolve, Polars’ commitment to performance, scalability, and user-friendliness positions it as a promising library for handling the complex and ever-expanding world of data. Whether you are dealing with small or large datasets, Polars offers a robust platform to meet your data analysis needs efficiently.
Pangaea X is a great resource for finding data analysts who are proficient in Polars. The platform has a large pool of freelancers who are experts in a variety of data science and analytics skills. Pangaea X can help you find the right freelancer to help you with your data analysis projects, regardless of the size or complexity of your data.
Get your data results fast and accelerate your business performance with the insights you need today.